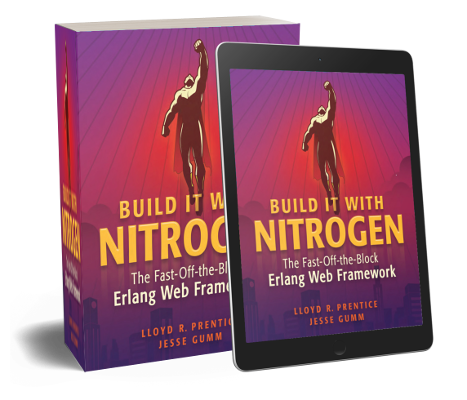
Build It With Nitrogen
The Fast-Off-the-Block Erlang Web Framework
By Lloyd R. Prentice and Jesse GummBuy Ebook
@ Leanpub Buy Paperback
@ Amazon
Download Sample Chapters
Are you a member of the media?
Build It with Nitrogen: the Fast Off the Block Erlang Web Framework guides web developers step-by-step through the construction of highly reliable web applications using Erlang and the Nitrogen Web Framework.
This easy-to-read book assumes minimal Linux or JavaScript skills; guides the reader through 7 hands-on projects. Each project builds on the last toward high-level competency. Readers learn Erlang as they go. Nitrogen simplifies development of web applications, making simple things easy and difficult things manageable.
Erlang delivers the high availability, massively scalable, soft real-time performance required by banking, e-commerce, computer telephony, and instant messaging applications.
What You'll Learn in Build It With Nitrogen
- How to build basic CRUD interfaces
- How to build highly dynamic interfaces
- How to work with the templates and the DOM
- How to work with logins and password hashing
- How to use OTP basics with gen_servers and supervisors
- How to use the databases: DETS, Mnesia, and PostgreSQL
- How to interact with external APIs (in our case, retrieving stock quotes)
- How to build your own behaviours and use those to produce Nitrogen pages more rapidly
- How to build custom Nitrogen elements and convert those into plugin libraries
- The basics of web application security
- The basics of using other front-end frameworks (specifically Bootstrap)
- How to avoid potential maintenance traps
- The basics of Git
- Learn Erlang along the way
Praise
"This book is ambitious. It covers not only Nitrogen and Erlang, but also a bit of OTP, databases, git, web design, and software engineering best practices. And somehow, it does this well by building up from simple concepts in a sort of 'Socratic dialogue'---if Socrates had a 'California surfer' sense of humor."
- Rusty Klophaus, creator Nitrogen
"This book is exactly what I wish existed when I started with Nitrogen and Erlang for the web. Takes you by the hand and explains Erlang, Nitrogen, databases and much more. Everything comes together to make your own fast dynamic website. Erlang and the web are a match made in heaven."
- Marc Worrell, creator Zotonic CMS
"... love it. 'Love it' is probably an under statement."
- Francesco Cesarini, Erlang Solutions
"Nitrogen simplifies development of web applications, making simple things easy and difficult things manageable. This book does the same. Projects present Nitrogen concepts and features in an iterative workflow that matches the way developers work. Developers will appreciate its many examples, its streamlined presentation, and numerous references to related material."
- Steve Vinoski, Yaws maintainer
"... a delightful and informative book that delivers the promise of the real-time web, combining colorful examples with battle-tested Erlang technology."
- Evan Miller, creator Chicago Boss
"An endless stream of real-world code samples on how to develop solid Erlang web applications with the Nitrogen framework. A real pleasure to read."
- Frank Müller, Erlang programmer
"I felt like the hero in an adventure story joining the characters and facing the challenges as a team. The fact that I learned about Nitrogen and web security was almost an after thought. I even learned how to extend Nitrogen with plugins! Serious learning wrapped in a fresh, fun package that kept me reading to the end."
- Alain O’Dea, Infrastructure and Security Manager, Sequence Bio
"...goes into great detail about Nitrogen and Erlang/OTP. Examples are simple yet functional with fictional dialogues bringing humor along the way. Nitrogen seems to be good for writing highly interactive Web applications entirely controlled from the server-side."
- Loïc Hoguin, creator Cowboy webserver
"Very fun book. Too many programming books are dry. Yours is just a joy to read."
- Alex Popov Jr.
Table of Contents
0.1 New to Erlang? . . . . . . . . . . . . . . . . . . . . . . . 3 I Frying Pan to Fire . . . . . . . . . . . . . . . . . . . . . . . . . 5 1 You Want Me to Build What? 7 2 Enter the Lion’s Den 9 2.1 The Big Picture . . . . . . . . . . . . . . . . . . . . . . . 10 2.2 Install Nitrogen . . . . . . . . . . . . . . . . . . . . . . 11 2.3 Lay of the Land . . . . . . . . . . . . . . . . . . . . . . . 13 2.4 Editors . . . . . . . . . . . . . . . . . . . . . . . . . . . 17 II If You Can’t Run, Dance! 21 3 nitroBoard I 23 3.1 Kill, Baby, Kill! . . . . . . . . . . . . . . . . . . . . . 23 3.2 Create a New Project . . . . . . . . . . . . . . . . . . . . 24 3.3 Prototype Welcome Page . . . . . . . . . . . . . . . . . . . 28 3.4 Anatomy of a Page . . . . . . . . . . . . . . . . . . . . . 31 3.5 Anatomy of a Route . . . . . . . . . . . . . . . . . . . . . 35 3.6 Anatomy of a Template . . . . . . . . . . . . . . . . . . . 36 3.7 Elements . . . . . . . . . . . . . . . . . . . . . . . . . . 36 3.8 Actions . . . . . . . . . . . . . . . . . . . . . . . . . . 39 3.9 Triggers and Targets . . . . . . . . . . . . . . . . . . . . 40 3.10 Enough Theory . . . . . . . . . . . . . . . . . . . . . . . 41 3.11 Visitors . . . . . . . . . . . . . . . . . . . . . . . . . . 45 3.12 Persistence . . . . . . . . . . . . . . . . . . . . . . . . 50 3.13 Styling . . . . . . . . . . . . . . . . . . . . . . . . . . 65 3.14 Debugging . . . . . . . . . . . . . . . . . . . . . . . . . 67 3.15 What You’ve Learned . . . . . . . . . . . . . . . . . . . . 68 3.16 Think and Do . . . . . . . . . . . . . . . . . . . . . . . . 68 4 nitroBoard II 4.1 Plan of Attack . . . . . . . . . . . . . . . . . . . . . . . 69 4.2 Associates . . . . . . . . . . . . . . . . . . . . . . . . . 70 4.3 I am in/I am out . . . . . . . . . . . . . . . . . . . . . . 79 4.4 Styling . . . . . . . . . . . . . . . . . . . . . . . . . . 83 4.5 What You’ve Learned . . . . . . . . . . . . . . . . . . . . 84 4.6 Think and Do . . . . . . . . . . . . . . . . . . . . . . . . 85 5 Nitrogen Functions 87 5.1 Wire Actions to the Browser . . . . . . . . . . . . . . . . 87 5.2 Retrieve Information Submitted by the User . . . . . . . . . 91 5.3 Change Elements on the Page . . . . . . . . . . . . . . . . 95 5.4 Conversion Functions . . . . . . . . . . . . . . . . . . . . 105 5.5 Encoding, Decoding, and Escaping . . . . . . . . . . . . . . 107 6 nindex 113 6.1 Oh CRUD . . . . . . . . . . . . . . . . . . . . . . . . . . 113 6.2 So Many DBs—So Little Time . . . . . . . . . . . . . . . . . 114 6.3 Lloyd London’s Weblink Application . . . . . . . . . . . . . 117 6.4 JoeDB . . . . . . . . . . . . . . . . . . . . . . . . . . . 120 6.5 Facade . . . . . . . . . . . . . . . . . . . . . . . . . . . 136 6.6 Search . . . . . . . . . . . . . . . . . . . . . . . . . . . 140 6.7 Enter the State Machine . . . . . . . . . . . . . . . . . . 144 6.8 State One: The first thing anyone sees . . . . . . . . . . . 145 6.9 State Two: Saving a New Weblink . . . . . . . . . . . . . . 147 6.10 State Three: Search . . . . . . . . . . . . . . . . . . . . 149 6.11 State Four: Display a Record . . . . . . . . . . . . . . . . 150 6.12 Page grid . . . . . . . . . . . . . . . . . . . . . . . . . 151 6.13 State One . . . . . . . . . . . . . . . . . . . . . . . . . 153 6.14 State Two: Add/Edit . . . . . . . . . . . . . . . . . . . . 155 6.15 State Three . . . . . . . . . . . . . . . . . . . . . . . . 160 6.16 Show all . . . . . . . . . . . . . . . . . . . . . . . . . . 163 6.17 Show Selected Link . . . . . . . . . . . . . . . . . . . . . 165 6.18 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . 173 7 nindex on Dets 175 7.1 Dets Again. . . No Wait, OTP. Yeah, OTP . . . . . . . . . . 175 7.2 Dets with OTP...For Real this Time . . . . . . . . . . . . . 206 8 nindex on PostgreSQL 225 8.1 Install PostgreSQL . . . . . . . . . . . . . . . . . . . . . 226 8.2 Create a Table . . . . . . . . . . . . . . . . . . . . . . . 230 8.3 Add the sql_bridge Dependency . . . . . . . . . . . . . . . 231 8.4 Configure sql_bridge . . . . . . . . . . . . . . . . . . . . 233 8.5 More on Maps . . . . . . . . . . . . . . . . . . . . . . . . 234 8.6 Build ni_pgsql with sql_bridge . . . . . . . . . . . . . . . 238 8.7 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . 244 9 nitroNotes 247 9.1 And So nnote Came to Be . . . . . . . . . . . . . . . . . . 247 9.2 Design—A Brief Aside . . . . . . . . . . . . . . . . . . . . 249 9.3 Static Grid . . . . . . . . . . . . . . . . . . . . . . . . 251 9.4 Create a New Project . . . . . . . . . . . . . . . . . . . . 252 9.5 Any Port in a Storm . . . . . . . . . . . . . . . . . . . . 253 9.6 Rusty’s Awesome Idea . . . . . . . . . . . . . . . . . . . . 254 9.7 A Matter of Style . . . . . . . . . . . . . . . . . . . . . 256 9.8 A Matter of Productivity . . . . . . . . . . . . . . . . . . 260 9.9 Main Menu . . . . . . . . . . . . . . . . . . . . . . . . . 263 9.10 Dare We Say Dynamic? . . . . . . . . . . . . . . . . . . . . 270 9.11 A Little Refactoring . . . . . . . . . . . . . . . . . . . . 281 9.12 Challenge! . . . . . . . . . . . . . . . . . . . . . . . . . 291 9.13 Oh Behave! . . . . . . . . . . . . . . . . . . . . . . . . . 294 9.14 Notes Notes and More Notes . . . . . . . . . . . . . . . . . 299 9.15 Datepicker . . . . . . . . . . . . . . . . . . . . . . . . . 309 10 nitroNotes II 315 10.1 Record Definitions . . . . . . . . . . . . . . . . . . . . . 317 10.2 create_id/0—Another Take . . . . . . . . . . . . . . . . . . 318 10.3 Speaking of mnesia . . . . . . . . . . . . . . . . . . . . . 310 10.4 mnesia Queries . . . . . . . . . . . . . . . . . . . . . . . 325 10.5 nnote Access Functions . . . . . . . . . . . . . . . . . . . 328 10.6 Stevie Takes a Flyer . . . . . . . . . . . . . . . . . . . . 333 10.7 Search and Select Functions . . . . . . . . . . . . . . . . 339 10.8 A bit about date handling . . . . . . . . . . . . . . . . . 340 10.9 Filter Functions Revisited . . . . . . . . . . . . . . . . . 342 10.10 Field Access Functions . . . . . . . . . . . . . . . . . . . 343 10.11 A Facade for nnote . . . . . . . . . . . . . . . . . . . . . 346 10.12 Add/Edit Form . . . . . . . . . . . . . . . . . . . . . . . 350 10.13 A Tricky Form . . . . . . . . . . . . . . . . . . . . . . . 353 10.14 Swing Back to Metadata . . . . . . . . . . . . . . . . . . . 362 10.15 Loose Ends . . . . . . . . . . . . . . . . . . . . . . . . . 367 10.16 Code Review with a Special Guest . . . . . . . . . . . . . . 376 10.17 Homework . . . . . . . . . . . . . . . . . . . . . . . . . . 402 11 Simple Login 405 11.1 Bird’s Eye View . . . . . . . . . . . . . . . . . . . . . . 405 11.2 More Things to Behave . . . . . . . . . . . . . . . . . . . 407 11.3 Registration Page . . . . . . . . . . . . . . . . . . . . . 411 11.4 Rebar Dependency: erlpass . . . . . . . . . . . . . . . . . 417 11.5 User Accounts . . . . . . . . . . . . . . . . . . . . . . . 421 11.6 Save the Day . . . . . . . . . . . . . . . . . . . . . . . . 432 11.7 Login! . . . . . . . . . . . . . . . . . . . . . . . . . . . 435 11.8 Ten Minutes Later . . . . . . . . . . . . . . . . . . . . . 439 11.9 Closing thoughts . . . . . . . . . . . . . . . . . . . . . . 445 11.10 Homework . . . . . . . . . . . . . . . . . . . . . . . . . . 446 12 But Is It Secure? 12.1 Trust No One! . . . . . . . . . . . . . . . . . . . . . . . 449 12.2 Hash Passwords . . . . . . . . . . . . . . . . . . . . . . . 449 12.3 Verify Logged-in Status in User-Specific Postbacks . . . . . 459 12.4 Hidden doesn’t mean gone . . . . . . . . . . . . . . . . . . 463 12.5 Conclusion and Recommendations for System-wide Security . . 465 III Going Further 469 13 Stockticker 471 13.1 Getting Started . . . . . . . . . . . . . . . . . . . . . . 472 13.2 Introducing httpc . . . . . . . . . . . . . . . . . . . . . 473 13.3 Bootstrap . . . . . . . . . . . . . . . . . . . . . . . . . 481 13.4 Stock Quotes . . . . . . . . . . . . . . . . . . . . . . . . 488 13.5 A menu for stockticker . . . . . . . . . . . . . . . . . . . 508 13.5 Stub in the Favorites Page . . . . . . . . . . . . . . . . . 516 13.6 Homework . . . . . . . . . . . . . . . . . . . . . . . . . . 517 14 Custom Elements . . . . . . . . . . . . . . . . . . . . . . . . . . 519 14.1 A Simple Element: #b{} . . . . . . . . . . . . . . . . . . . 519 14.2 Advanced Custom Element #time_selector{} . . . . . . . . . . 526 14.3 X-Treme #time_selector{}! Custom Postbacks . . . . . . . . . 532 15 Nitrogen Plugins 537 15.1 So Now in a Crufty Booth . . . . . . . . . . . . . . . . . . . 537 16 The Wide World is Waiting 543 IV Appendices 545 A Erlang from the Top Down 547 B Erlang Resources 555 C Just Enough Git 557